x |
y |
goal(x, y) |
1 |
1 |
0 |
1 |
0 |
1 |
0 |
1 |
0 |
0 |
0 |
0 |
(These tables are just stock Obsidian tables copied and pasted from my note and they render perfectly. cool!)
3 false and 1 true input scenario. The true input scenario is:
(x ^ ~y)
Correct
x |
y |
goal-2(x, y) |
1 |
1 |
1 |
1 |
0 |
1 |
0 |
1 |
0 |
0 |
0 |
0 |
two true input scenarios:
- (x^y)
- (x^~y)
so (x^y)v(x^~y) is an equivalent expression for goal-2(x, y)
goal-2(x, y) = (x^y)v(x^~y)
also
goal-2(x, y) = (x)
i.e just (x) is equivalent to goal-2(x, y)
x |
y |
goal-3(x, y) |
1 |
1 |
1 |
1 |
0 |
0 |
0 |
1 |
1 |
0 |
0 |
1 |
So there are 3 true input cases. To make it simple, if I negate the false input case, itāll imply the three true input cases. So:
goal-3(x, y) = ~(x^~y)
I know Iām mixing up prefix and infix notation and thatās not ideal.
False input scenarios:
so we know that:
goal-3(x, y) = ~(x^~y)
What this means is: goal-3 is true when itās not the case that x is true and y is false. So what is goal-3 when it is the case that x is true and y is false? In that case, goal-3 is false:
~goal-3(x, y) = (x^~y)
This means what we just said which is that goal-3 is false when x is true and y is false. So the negation of the case equivalent to goal-3 being true, is the equivalent to the case of goal-3 being false.
okay so i.e what is the expression for:
x |
y |
goal-false(x, y) |
1 |
1 |
0 |
1 |
0 |
0 |
0 |
1 |
0 |
0 |
0 |
0 |
you could specify and then negate all the false input scenarios:
goal-false(x, y) = ~((x^y)v(x^~y)v(~x^y)v(~x^~y))
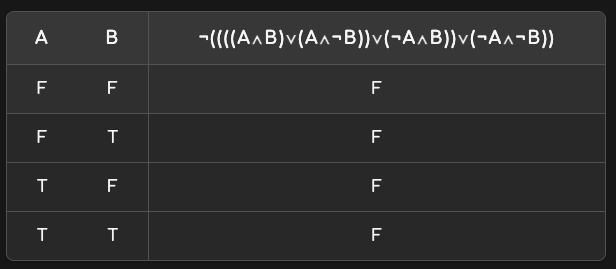
This means: goal-false(x, y) is true when there is an input scenario other than the four possible input scenarios, which since impossible is never, so itās always false.
You could do simpler though:
goal-false(x, y) = ~(xv~x)
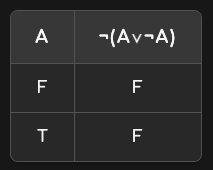
Which means: goal-false is true when itās not the case that x is true or not x is true. Thatāll mean that goal-false is never true because in all input scenarios x is either true or false. So ~(xv~x) always outputs false like goal-false(x, y). ~(yv~y) also works for the same reason.
I feel like Iāve made some good progress on my problem with understanding the concepts and I notice it feels great. Like Iāve really enjoyed studying and practising today more than other days and Iāve spent more time doing it over what I scheduled/expected.